Introduction
When working with strings in JavaScript, it is often necessary to check if a string contains a specific substring. Whether you need to validate user input, search for keywords, or perform conditional logic based on the presence of a substring, JavaScript provides several methods and techniques to accomplish this task. In this article, we will explore various approaches to check if a string contains a substring in JavaScript.

Using the indexOf() Method
One of the most common and straightforward ways to check for the presence of a substring in a string is by using the indexOf()
method. This method returns the index of the first occurrence of the substring within the string. If the substring is not found, it returns -1. By checking the returned value against -1, we can determine if the string contains the desired substring.
Using the includes() Method
Introduced in ECMAScript 2015 (ES6), the includes()
method provides a more concise and expressive way to check if a string contains a substring. This method returns a boolean value indicating whether the substring is found within the string. Unlike the indexOf()
method, it does not return the index of the substring. Instead, it directly informs us if the substring exists in the string.
Using Regular Expressions
Regular expressions offer powerful pattern-matching capabilities, making them a versatile tool for string manipulation. In JavaScript, we can use regular expressions to check if a string contains a specific substring. By creating a regular expression pattern that matches the desired substring and using methods such as test()
or match()
, we can determine if the string contains the substring based on the pattern matching result.
Using the search() Method
The search()
method is another option to check if a string contains a substring using regular expressions. This method searches for a specified pattern (substring) within the string and returns the index of the first occurrence. If the pattern is not found, it returns -1. Similar to the indexOf()
method, we can use the returned value to determine if the substring exists in the string.
Using the match() Method
The match()
method, when combined with regular expressions, allows us to extract the matching parts of a string. By using a regular expression pattern that matches the desired substring and invoking the match()
method, we can retrieve an array containing the matched substring(s). If the array is not empty, it indicates that the substring exists in the string.
Performance Considerations
When dealing with large strings or performing frequent substring checks, it’s important to consider performance. Some methods, such as indexOf()
and includes()
, are generally more efficient than others, such as regular expressions. However, the actual performance may vary depending on the specific use case and the length of the string. It’s advisable to benchmark and test different approaches to determine the most suitable solution for your scenario.
Case Sensitivity
By default, the methods we discussed are case-sensitive, meaning they distinguish between uppercase and lowercase letters. If you want to perform a case-insensitive search, you can convert both the string and the substring to either lowercase or uppercase using methods like toLowerCase()
or toUpperCase()
. This ensures that the comparison is not affected by letter case.
Conclusion
Checking if a string contains a substring is a fundamental operation in JavaScript programming. By leveraging methods like indexOf()
, includes()
, and regular expressions, we can easily determine whether a string contains a specific substring. Consider the nature of your task, performance requirements, and case sensitivity when choosing the appropriate approach. With these techniques in your toolkit, you can effectively handle substring checks and perform various string manipulation tasks in JavaScript.

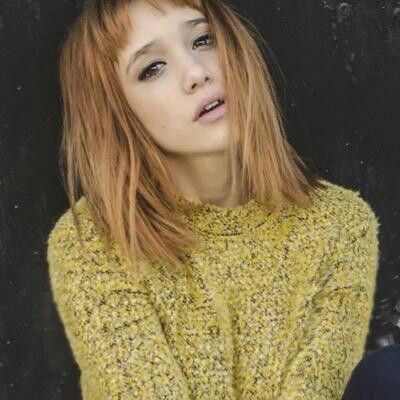
Hi all, my name is Angelika and I am one of the authors of the EasyTechh website. Like the rest of our team I am incredibly ambitious and I love helping people.
That’s why I write here and not only here ๐ I write interesting and useful for people articles in the IT sphere and a little bit about life.
Enjoy reading.
+ There are no comments
Add yours