Introduction to Python Dictionaries
Python dictionaries are versatile data structures that allow you to store key-value pairs. They are an essential tool in Python programming and provide efficient lookup and retrieval of values based on their keys. In this article, we will explore how to create dictionaries in Python and learn about various dictionary methods and operations. By the end of this article, you will have a solid understanding of dictionaries and be able to use them effectively in your Python code.
Creating a Dictionary in Python
Overview of Dictionaries
A dictionary in Python is created using curly braces {}
and consists of key-value pairs separated by colons :
. The keys are unique and can be of any immutable data type, such as strings, numbers, or tuples. The corresponding values can be of any data type, including lists, tuples, dictionaries, or even custom objects.
Example of Creating a Dictionary
Let’s start with a simple example to create a dictionary in Python:
student = {
"name": "John Doe",
"age": 20,
"grade": "A"
}
In this example, we have created a dictionary named student
with three key-value pairs. The keys are "name"
, "age"
, and "grade"
, and the corresponding values are "John Doe"
, 20
, and "A"
.
Dictionary Methods and Operations
Python provides a rich set of built-in methods and operations to work with dictionaries. Let’s explore some of the commonly used ones:
Adding and Updating Elements
You can add new key-value pairs to a dictionary or update existing values using the assignment operator =
. If the key already exists, the value will be updated; otherwise, a new key-value pair will be added.
student = {
"name": "John Doe",
"age": 20
}
# Adding a new key-value pair
student["grade"] = "A"
# Updating an existing value
student["age"] = 21
print(student)
Output:
{
"name": "John Doe",
"age": 21,
"grade": "A"
}
In this example, we add a new key-value pair "grade": "A"
to the student
dictionary and update the value of the "age"
key to 21
.
Accessing Values
You can retrieve the value associated with a key in a dictionary using the square bracket notation []
. If the key doesn’t exist, a KeyError
will be raised. To avoid this, you can use the get()
method, which returns None
or a default value if the key is not found.
student = {
"name": "John Doe",
"age": 20,
"grade": "A"
}
# Accessing values using square brackets
print(student["name"]) # Output: John Doe
# Accessing values using get()
print(student.get("age")) # Output: 20
# Accessing a non-existent key
print(student.get("address")) # Output: None
In this example, we retrieve the values associated with the "name"
and "age"
keys using both the square bracket notation and the get()
method. We also demonstrate accessing a non-existent key, which returns None
instead of raising an error.
Removing Elements
You can remove key-value pairs from a dictionary using the del
statement or the pop()
method. The del
statement removes the key-value pair directly, while the pop()
method removes the specified key and returns its value.
student = {
"name": "John Doe",
"age": 20,
"grade": "A"
}
# Removing a key-value pair using del
del student["age"]
# Removing a key-value pair using pop()
grade = student.pop("grade")
print(student)
print(grade)
Output:
{
"name": "John Doe"
}
A
In this example, we remove the key-value pair "age": 20
using the del
statement and remove the key "grade"
and retrieve its value using the pop()
method.
Other Dictionary Methods
Python dictionaries offer additional methods to perform various operations, such as checking for the existence of a key, retrieving all keys or values, and clearing the dictionary. Some commonly used methods include:
keys()
: Returns a list of all the keys in the dictionary.values()
: Returns a list of all the values in the dictionary.items()
: Returns a list of all the key-value pairs as tuples.clear()
: Removes all key-value pairs from the dictionary.
student = {
"name": "John Doe",
"age": 20,
"grade": "A"
}
# Getting all keys
keys = student.keys()
# Getting all values
values = student.values()
# Getting all
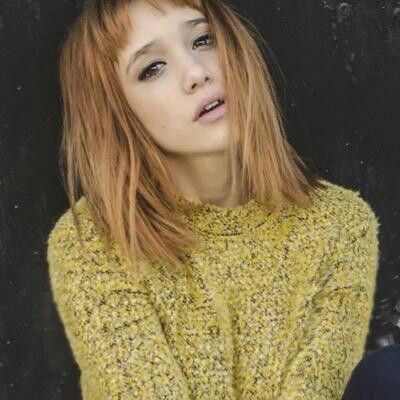
Hi all, my name is Angelika and I am one of the authors of the EasyTechh website. Like the rest of our team I am incredibly ambitious and I love helping people.
That’s why I write here and not only here ๐ I write interesting and useful for people articles in the IT sphere and a little bit about life.
Enjoy reading.
+ There are no comments
Add yours