Introduction to Printing Variables in Python
Printing variables is a fundamental skill in Python programming. It allows you to display the values stored in variables and track the state of your program during execution. In this article, we will explore different techniques to print variables in Python and discuss their usage. By the end of this article, you will have a clear understanding of how to print variables effectively in Python.
Printing Variables using the print()
Function
Overview of the print()
Function
The print()
function is a built-in function in Python that outputs a specified message or value to the console. It is commonly used to print variables and display information during program execution.
Syntax of the print()
Function
The syntax for the print()
function is as follows:
print(value1, value2, ..., sep=' ', end='\n', file=sys.stdout, flush=False)
The print()
function accepts one or more values as arguments and displays them on the console. By default, the values are separated by a space character (sep=' '
) and followed by a newline character (end='\n'
). However, you can customize the separator and end character as per your requirements.
Example of Printing Variables using the print()
Function
Here’s an example that demonstrates how to print variables using the print()
function:
name = "John"
age = 25
print("Name:", name)
print("Age:", age)
Output:
Name: John
Age: 25
In this example, we define two variables name
and age
. We then use the print()
function to display the values of these variables along with descriptive labels.
Printing Variables with Formatted Strings
Overview of Formatted Strings
Formatted strings, also known as f-strings, provide a concise and readable way to format and print variables in Python. They allow you to embed variables directly within a string, making the code more expressive and easier to understand.
Syntax of Formatted Strings
The syntax for formatted strings is as follows:
f"string {variable}"
You can include variables inside curly braces {}
within the string and prefix the string with the letter ‘f’ to indicate that it is a formatted string.
Example of Printing Variables with Formatted Strings
Here’s an example that demonstrates how to print variables using formatted strings:
name = "John"
age = 25
print(f"Name: {name}")
print(f"Age: {age}")
Output
Name: John
Age: 25
In this example, we use formatted strings to embed the variables name
and age
directly within the print statements. The values of the variables are automatically substituted within the string.
Printing Variables with String Concatenation
Overview of String Concatenation
String concatenation is the process of combining strings together. It can be used to print variables by concatenating them with other string literals or variables.
Example of Printing Variables with String Concatenation
Here’s an example that demonstrates how to print variables using string concatenation:
name = "John"
age = 25
print("Name: " + name)
print("Age: " + str(age))
Output:
Name: John
Age: 25
In this example, we concatenate the variables name
and age
with the string literals using the +
operator. The str()
function is used to convert the integer variable age
into a string before concatenation.
Conclusion
Printing variables is a crucial skill in Python programming. Whether you use the print()
function, formatted strings, or string concatenation, it’s important to choose the method that best suits your needs and enhances the readability of your code. By mastering the techniques discussed in this article, you will be able to effectively print variables and display information in your Python programs.
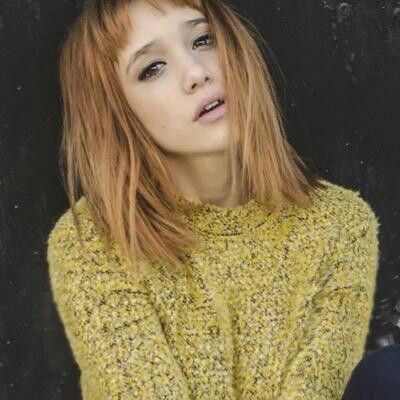
Hi all, my name is Angelika and I am one of the authors of the EasyTechh website. Like the rest of our team I am incredibly ambitious and I love helping people.
That’s why I write here and not only here ๐ I write interesting and useful for people articles in the IT sphere and a little bit about life.
Enjoy reading.
+ There are no comments
Add yours