Introduction
Sending HTTP requests is a fundamental aspect of web development, and JavaScript provides several methods to achieve this. One of the commonly used methods is sending an HTTP POST request. In this article, we will explore how to send an HTTP POST request using JavaScript. We will cover the basic concepts of POST requests, examine the different approaches in JavaScript, and provide practical examples to help you understand and implement this functionality in your projects.
Understanding HTTP POST Requests
When performing HTTP requests, there are typically two types: GET and POST. While a GET request is used to retrieve data from a server, a POST request is used to send data to a server for processing. POST requests are commonly used when submitting forms, uploading files, or performing any action that requires sending data to a server.
Sending an HTTP POST Request in JavaScript
JavaScript provides different methods for sending an HTTP POST request. Let’s explore three popular approaches:
XMLHttpRequest
The XMLHttpRequest
object is a traditional way to send HTTP requests in JavaScript. It provides a low-level interface for making asynchronous requests. Here’s an example of how to send an HTTP POST request using XMLHttpRequest
:
const xhr = new XMLHttpRequest();
const url = "https://example.com/api/data";
const data = { name: "John", age: 25 };
xhr.open("POST", url, true);
xhr.setRequestHeader("Content-Type", "application/json");
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
// Request completed successfully
console.log(xhr.responseText);
}
};
xhr.send(JSON.stringify(data));
In this example, we create a new XMLHttpRequest
object, specify the request URL, set the request method to POST, and set the request header to indicate the content type as JSON. We then define a callback function to handle the response when the request is complete. Finally, we send the request with the serialized JSON data.
Fetch API
The Fetch API is a modern and more flexible approach to making HTTP requests in JavaScript. It provides a simpler and more promise-based syntax compared to XMLHttpRequest
. Here’s an example of how to send an HTTP POST request using the Fetch API:
const url = "https://example.com/api/data";
const data = { name: "John", age: 25 };
fetch(url, {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => {
// Request completed successfully
console.log(data);
})
.catch(error => {
// Error occurred during the request
console.error(error);
});
In this example, we use the fetch
function to send an HTTP POST request. We pass the request URL, method, headers, and the serialized JSON data as the request body. We then handle the response by parsing it as JSON and accessing the data. In case of an error, we catch and handle it accordingly.
Axios Library
Axios is a popular JavaScript library for making HTTP requests. It provides a simple and elegant API for performing AJAX requests. To use Axios, you need to include it in your project by either downloading it or using a CDN. Here’s an example of how to send an HTTP POST request using Axios:
const url = "https://example.com/api/data";
const data = { name: "John", age: 25 };
axios.post(url, data)
.then(response => {
// Request completed successfully
console.log(response.data);
})
.catch(error => {
// Error occurred during the request
console.error(error);
});
In this example, we use the axios.post
method to send an HTTP POST request. We provide the request URL and the data object as arguments. Axios automatically serializes the data as JSON and sets the appropriate headers. We handle the response and any errors using the promise-based syntax.
Conclusion
Sending an HTTP POST request in JavaScript is a crucial skill for web developers. Whether you’re interacting with APIs, submitting form data, or performing server-side actions, understanding how to send POST requests is essential. In this article, we explored three different approaches: XMLHttpRequest
, the Fetch API, and the Axios library. Each approach has its advantages and suitability based on your project’s requirements. Experiment with these techniques and choose the one that best fits your needs. By mastering HTTP POST requests in JavaScript, you can enhance the interactivity and functionality of your web applications.
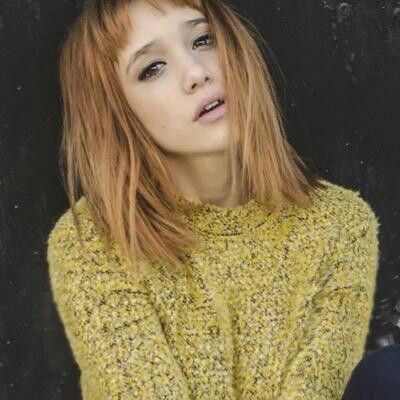
Hi all, my name is Angelika and I am one of the authors of the EasyTechh website. Like the rest of our team I am incredibly ambitious and I love helping people.
That’s why I write here and not only here ๐ I write interesting and useful for people articles in the IT sphere and a little bit about life.
Enjoy reading.
+ There are no comments
Add yours