Introduction
In JavaScript, executing code when the Document Object Model (DOM) is ready is a common requirement. The document.ready()
function in jQuery provides a convenient way to ensure that your JavaScript code is executed only after the DOM has fully loaded. This article will guide you through the usage of document.ready()
in jQuery and provide examples to help you understand its practical implementation.
Understanding the Document Ready Event
The Document Ready event occurs when the DOM has finished loading and is ready to be manipulated. It ensures that your JavaScript code is executed at the appropriate time, preventing any issues that may arise from accessing DOM elements before they are fully loaded. By using the document.ready()
function, you can guarantee that your code will run only after the DOM is ready, providing a smooth and error-free user experience.
Using document.ready() in jQuery
To utilize the document.ready()
function in jQuery, follow these simple steps:
Step 1: Include the jQuery Library
First, make sure to include the jQuery library in your HTML file. You can either download jQuery and host it locally or use a Content Delivery Network (CDN) to include it directly in your project. Here’s an example of including jQuery using a CDN:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 2: Wrap Your Code in document.ready()
Once jQuery is included, you can wrap your JavaScript code inside the document.ready()
function. This ensures that the code will be executed only when the DOM is ready. Here’s an example:
$(document).ready(function() {
// Your code here
});
Alternatively, you can use the shorthand notation $()
instead of $(document).ready()
. Here’s the equivalent code using the shorthand notation:
$(function() {
// Your code here
});
Step 3: Write Your JavaScript Code
Inside the document.ready()
function, you can write your JavaScript code that interacts with the DOM. This code will be executed once the DOM is fully loaded and ready for manipulation. For example, you can access and modify HTML elements, bind event handlers, or perform any other operations involving the DOM.
Example Usage
Let’s look at an example to illustrate the usage of document.ready()
in jQuery. Suppose we want to change the text of a paragraph element with the ID “myParagraph” when the DOM is ready. Here’s how the code would look:
<!DOCTYPE html>
<html>
<head>
<title>Document Ready Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<p id="myParagraph">Original Text</p>
<script>
$(function() {
$("#myParagraph").text("New Text");
});
</script>
</body>
</html>
In this example, the paragraph element with the ID “myParagraph” will have its text changed to “New Text” once the DOM is ready.
Conclusion
The document.ready()
function in jQuery is a powerful tool for ensuring that your JavaScript code is executed only after the DOM has finished loading. By using this function, you can avoid errors caused by accessing DOM elements prematurely. Incorporating document.ready()
into your JavaScript code enhances the reliability and performance of your web applications. Experiment with the examples provided and explore further possibilities to leverage the capabilities of document.ready()
in your own projects.
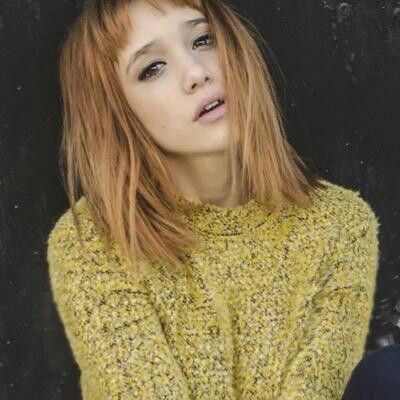
Hi all, my name is Angelika and I am one of the authors of the EasyTechh website. Like the rest of our team I am incredibly ambitious and I love helping people.
That’s why I write here and not only here ๐ I write interesting and useful for people articles in the IT sphere and a little bit about life.
Enjoy reading.
+ There are no comments
Add yours