Introduction
The clipboard is a crucial feature in mobile devices that allows users to copy and paste text, images, and other data across different applications. Android provides a convenient way to access the clipboard programmatically and perform operations such as reading and clearing its contents. In this article, we will explore how to access the clipboard in Android and clear its contents programmatically.
Accessing the Clipboard in Android
To access the clipboard in Android, we can make use of the ClipboardManager
class, which is available in the Android framework. Here’s how you can access the clipboard:
// Get the ClipboardManager instance
ClipboardManager clipboardManager = (ClipboardManager) getSystemService(Context.CLIPBOARD_SERVICE);
By obtaining the ClipboardManager
instance, we can now perform various operations on the clipboard.
Reading Text from the Clipboard
To read text from the clipboard, you can use the getPrimaryClip()
method of the ClipboardManager
class. Here’s an example:
// Get the primary clip from the clipboard
ClipData clipData = clipboardManager.getPrimaryClip();
// Check if the clipboard contains text
if (clipData != null && clipData.getItemCount() > 0) {
// Get the text from the first item in the clip data
CharSequence text = clipData.getItemAt(0).getText();
// Do something with the text
// ...
}
In this example, we check if the clipboard contains any text, and if so, we retrieve the text from the first item in the clip data.
Writing Text to the Clipboard
To write text to the clipboard, you can use the setText()
method of the ClipboardManager
class. Here’s an example:
// Create a new ClipData object with the text
ClipData clipData = ClipData.newPlainText("label", "Text to copy to clipboard");
// Set the clip data to the clipboard
clipboardManager.setPrimaryClip(clipData);
In this example, we create a new ClipData
object with the desired text and set it as the primary clip in the clipboard.
Clearing the Clipboard
To clear the contents of the clipboard, you can use the clearPrimaryClip()
method of the ClipboardManager
class. Here’s an example:
// Clear the contents of the clipboard
clipboardManager.clearPrimaryClip();
By calling this method, you remove any data that is currently stored in the clipboard.
Checking Clipboard Availability
Before performing any clipboard operations, it’s a good practice to check if the clipboard is available. You can use the hasPrimaryClip()
method of the ClipboardManager
class to determine if the clipboard has any data. Here’s an example:
// Check if the clipboard has primary clip data
boolean hasClipData = clipboardManager.hasPrimaryClip();
By checking the result of this method, you can ensure that the clipboard is accessible before attempting to read or modify its contents.
Conclusion
Accessing and manipulating the clipboard in Android is essential for providing a seamless user experience. By using the ClipboardManager
class, you can easily read text from the clipboard, write text to it, and clear its contents. Remember to handle any potential exceptions that may occur when accessing the clipboard and perform necessary checks to ensure its availability.
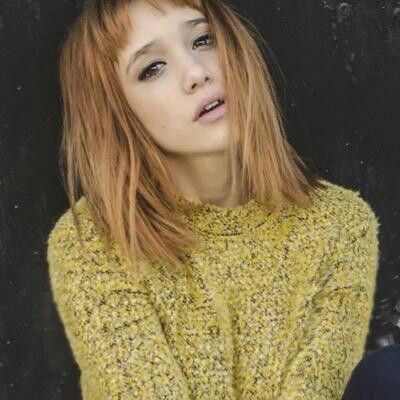
Hi all, my name is Angelika and I am one of the authors of the EasyTechh website. Like the rest of our team I am incredibly ambitious and I love helping people.
That’s why I write here and not only here ๐ I write interesting and useful for people articles in the IT sphere and a little bit about life.
Enjoy reading.
+ There are no comments
Add yours